How do you generate a random number between 1 and 10 in Python?
Use a random.randint() function to get a random integer number from the inclusive range. For example, random.randint(0, 10) will return a random number from [0, 1, 2, 3, 4, 5, 6, 7, 8 ,9, 10].
How do you generate a random number from 1 to 10?
Using random. nextInt() to generate random number between 1 and 10
- randomGenerator.nextInt((maximum – minimum) + 1) + minimum. In our case, minimum = 1. maximum = 10so it will be.
- randomGenerator.nextInt((10 – 1) + 1) + 1.
- randomGenerator.nextInt(10) + 1.
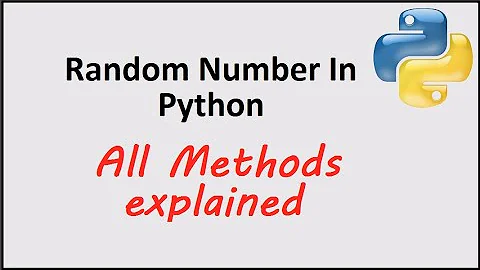
How do you generate random 10 numbers in Python?
Generate random number between 1 and 10 in Python
- Using the random.randint() function.
- Using the random.randrange() function.
- Using the random.sample() function.
- Using the random.uniform() function.
- Using the numpy.random.randint() function.
- Using the numpy.random.uniform() function.
- Using the numpy.random.choice() function.
How do you make a 1 to 10 in Python?
Python: Generate and prints a list of numbers from 1 to 10
- Sample Solution:
- Python Code: nums = range(1,10) print(list(nums)) print(list(map(str, nums))) …
- Flowchart:
- Python Code Editor: …
- Have another way to solve this solution? …
- Previous: Write a Python program to print letters from the English alphabet from a-z and A-Z.
How do you generate a random number between 1 and 6 in Python?
Generating a random integer within a given range – randint()
- Firstly, import the random module of Python.
- Then, we call the randint(min, max) method to get a random integer within the given range and store it in the variable n.
- Print the randomly generated number.
What is the best number to pick between 1 and 10?
7
It's well known amongst purveyors of conjuring tricks and the like that if you ask people to pick a number between 1 and 10, far more people choose 7 than any other number.
What are the numbers between 1 and 10?
First of all let us consider all the numbers from 1 to 10 that are 1, 2, 3, 4, 5, 6, 7, 8, 9, and 10. Here, we already know that the number 1 is not considered as prime or composite.
How do you generate random numbers in Python?
To generate random number in Python, randint() function is used. This function is defined in random module.
How do you generate a random number in Python example?
Python random module provides the randint() method that generates an integer number within a specific range.
…
Generating a Number within a Given Range
- import random.
- n = random. randint(0,50)
- print(n)
How do you make a number between 1 to 20 in Python?
Python Program to Generate Random Numbers from 1 to 20 and Append Them to the List
- Import the random module into the program.
- Take the number of elements from the user.
- Use a for loop, random. randint() is used to generate random numbers which are them appending to a list.
- Then print the randomised list.
How do you generate a random number from 1 to 100 in Python?
Generate a random number between 1 and 100
x = randint(1, 100) # Pick a random number between 1 and 100.
How do you generate a number from 1 to 100 in Python?
Using the range() function to create a list from 1 to 100 in Python. In Python, we can use the range() function to create an iterator sequence between two endpoints. We can use this function to create a list from 1 to 100 in Python. The function accepts three parameters start , stop , and step .
What is the most common random number between 1 and 10?
According to the video, if you ask people to randomly pick any one integer between 1 and 10 (both inclusive), people are more likely to choose 7.
How do you do the pick a number between 1 and 10 trick?
Pick a whole number between 1 and 10.
…
Trick 2: Think of a number
- Choose a number from 1 to 8.
- Multiply it by 2.
- Now multiply by 5.
- Subtract 5.
- Finally add 7.
- The first digit is the number you chose and the second digit is the number 2.
What is random random () Python?
Python Random random() Method
The random() method returns a random floating number between 0 and 1.
How do you generate a random number?
There are two common methods for generating random numbers from a computer: Pseudo-Random Number Generators (PRNGs) and True Random Number Generators (TRNGs). To generate PRNGs, a computer uses a seed number and an algorithm to generate numbers that seem to be random, but are actually predictable.
How does random () work Python?
The random() method returns a random floating number between 0 and 1.
What is random () in Python?
- random() function generates random floating numbers in the range[0.1, 1.0). (See the opening and closing brackets, it means including 0 but excluding 1). It takes no parameters and returns values uniformly distributed between 0 and 1.
How do you generate numbers from 1 to 100 in Python?
Using the range() function to create a list from 1 to 100 in Python. In Python, we can use the range() function to create an iterator sequence between two endpoints. We can use this function to create a list from 1 to 100 in Python. The function accepts three parameters start , stop , and step .
How do you set a random number in Python?
- To generate random number in Python, randint() function is used. This function is defined in random module.
What is the use of the pick random 1 to 10 block?
The pick random operator allows you to generate random numbers in your programs. When you use the block you need to set the range of numbers that Scratch will choose from. In the example shown, Scratch will pick a number between (and including) 1 and 10.
How Python generate random numbers?
To generate random number in Python, randint() function is used. This function is defined in random module.
How do I get a random number in Python?
To generate random number in Python, randint() function is used. This function is defined in random module.
How does random work in Python?
The random. randrange() method returns a randomly selected element from the range created by the start, stop and step arguments. The value of start is 0 by default. Similarly, the value of step is 1 by default.
How does Python pick a random number?
Use a random.randint() function to get a random integer number from the inclusive range. For example, random.randint(0, 10) will return a random number from [0, 1, 2, 3, 4, 5, 6, 7, 8 ,9, 10].
What does the random () method do?
random() is used to return a pseudorandom double type number greater than or equal to 0.0 and less than 1.0. The default random number always generated between 0 and 1. If you want to specific range of values, you have to multiply the returned value with the magnitude of the range.