How to break the loop in C?
In C, if you want to exit a loop when a specific condition is met, you can use the break statement. As with all statements in C, the break statement should terminate with a semicolon ( ; ).
How do you break a loop?
Tips
- The break statement exits a for or while loop completely. To skip the rest of the instructions in the loop and begin the next iteration, use a continue statement.
- break is not defined outside a for or while loop. To exit a function, use return .
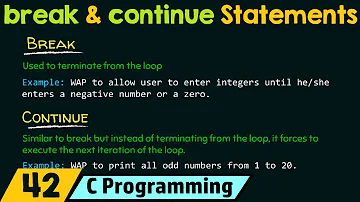
How to break infinite loop in C?
In order to come out of the infinite loop, we can use the break statement.
…
while loop
- while(1)
- {
- // body of the loop..
- }
How to use exit () in C?
C library function – exit()
The C library function void exit(int status) terminates the calling process immediately. Any open file descriptors belonging to the process are closed and any children of the process are inherited by process 1, init, and the process parent is sent a SIGCHLD signal.
How to break nested loops in C?
The break is a keyword in C which is used to bring the program control out of the loop. The break statement is used inside loops or switch statement. The break statement breaks the loop one by one, i.e., in the case of nested loops, it breaks the inner loop first and then proceeds to outer loops.
What is break in C language?
The break statement terminates the execution of the nearest enclosing do , for , switch , or while statement in which it appears. Control passes to the statement that follows the terminated statement.
How do you break a repeat loop?
A repeat loop is used to iterate over a block of code multiple number of times. There is no condition check in repeat loop to exit the loop. The only way to exit a repeat loop is to call break. These are not commonly used in statistical or data analysis applications but they do have their uses.
Does Break exit all loops C?
The break in C is a loop control statement that terminates a loop when encountered.
What is the break statement in C?
The break statement terminates the execution of the nearest enclosing do , for , switch , or while statement in which it appears. Control passes to the statement that follows the terminated statement.
Is there break in C?
break command (C and C++)
The break command allows you to terminate and exit a loop (that is, do , for , and while ) or switch command from any point other than the logical end. You can place a break command only in the body of a looping command or in the body of a switch command.
What is exit () function?
The exit() function is used to terminate a process or function calling immediately in the program. It means any open file or function belonging to the process is closed immediately as the exit() function occurred in the program.
Does break exit all loops in C?
When a break statement is encountered inside a loop, the loop is immediately terminated and the program control resumes at the next statement following the loop.
How do you exit a nested loop?
Conclusion
The break keyword is helpful for single loops, and we can use labeled breaks for nested loops. Alternatively, we can use a return statement.
Does break stop all loops?
The break in C is a loop control statement that terminates a loop when encountered. It can be used inside loops or switch statements to bring the control out of the block. The break statement can only break out of a single loop at a time.
What is break and continue in C?
The primary difference between break and continue statement in C is that the break statement leads to an immediate exit of the innermost switch or enclosing loop. On the other hand, the continue statement begins the next iteration of the while, enclosing for, or do loop.
Can you break out of two loops?
BREAK will only break out of the loop in which it was called. As a workaround, you can use a flag variable along with BREAK to break out of nested loops.
Can you break for loops?
Breaking Out of For Loops. To break out of a for loop, you can use the endloop, continue, resume, or return statement.
How do you stop a while loop?
- To break out of a while loop, you can use the endloop, continue, resume, or return statement. endwhile; If the name is empty, the other statements are not executed in that pass through the loop, and the entire loop is closed.
What is break continue in C?
The primary difference between break and continue statement in C is that the break statement leads to an immediate exit of the innermost switch or enclosing loop. On the other hand, the continue statement begins the next iteration of the while, enclosing for, or do loop.
What is break Control in C?
- The break statement terminates the execution of the nearest enclosing do , for , switch , or while statement in which it appears. Control passes to the statement that follows the terminated statement.
What does break do in C ++?
break Statement (C++)
The break statement ends execution of the nearest enclosing loop or conditional statement in which it appears. Control passes to the statement that follows the end of the statement, if any.
How to stop a code in C?
So the C family has three ways to end the program: exit(), return, and final closing brace.
How do you break out of two loops?
Breaking out of two loops
- Put the loops into a function, and return from the function to break the loops. …
- Raise an exception and catch it outside the double loop. …
- Use boolean variables to note that the loop is done, and check the variable in the outer loop to execute a second break.
How do you exit a while loop in C?
break command (C and C++)
The break command allows you to terminate and exit a loop (that is, do , for , and while ) or switch command from any point other than the logical end. You can place a break command only in the body of a looping command or in the body of a switch command.
Can you break while loops?
Breaking Out of While Loops. To break out of a while loop, you can use the endloop, continue, resume, or return statement. endwhile; If the name is empty, the other statements are not executed in that pass through the loop, and the entire loop is closed.
Does break end all loops in C?
break command (C and C++)
break ; In a looping statement, the break command ends the loop and moves control to the next command outside the loop. Within nested statements, the break command ends only the smallest enclosing do , for , switch , or while commands.