How is the array declared in a JavaScript?
Creating an Array Using an array literal is the easiest way to create a JavaScript Array. Syntax: const array_name = [item1, item2, …]; It is a common practice to declare arrays with the const keyword.
How is an array declared?
Declaring Arrays
Array variables are declared identically to variables of their data type, except that the variable name is followed by one pair of square [ ] brackets for each dimension of the array. Uninitialized arrays must have the dimensions of their rows, columns, etc. listed within the square brackets.
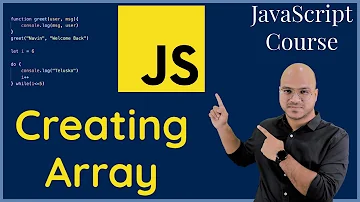
How to declare array of objects in JavaScript?
To create an array of objects in javascript, we have to declare an empty array first and then initialize it with the objects separated by a comma.
How do we declare and initialize an array in JavaScript?
How to Declare and Initialize an Array in JavaScript
- let x = new Array(); – an empty array.
- let x = new Array(10,20,30); – three elements in the array: 10,20,30.
- let x = new Array(10); – ten empty elements in array: ,,,,,,,,,
- let x = new Array('10'); – an array with 1 element: '10'
How to declare list in JavaScript?
To create a list of objects in JavaScript, utilize the “for” loop method to iterate along the list objects with the help of the length property, the “forEach()” method to relate the newly created objects with the array values and append it to a new list, or the “map()” method to map the function on the created array in …
How is an array declared and initialized?
The initializer for an array is a comma-separated list of constant expressions enclosed in braces ( { } ). The initializer is preceded by an equal sign ( = ). You do not need to initialize all elements in an array.
What are the two ways to declare an array?
There are two ways you can declare and initialize an array in Java. The first is with the new keyword, where you have to initialize the values one by one. The second is by putting the values in curly braces.
How to declare array of array in JavaScript?
To declare an array with literal notation you just define a new array using empty brackets. It looks like this: let myArray = []; You will place all elements within the square brackets and separate each item or element with a comma.
What is array in JavaScript with example?
An array is an object that can store multiple values at once. For example, const words = ['hello', 'world', 'welcome']; Here, words is an array.
How do you declare and create an array?
To create an array, define the data type (like int ) and specify the name of the array followed by square brackets []. To insert values to it, use a comma-separated list, inside curly braces: int myNumbers[] = {25, 50, 75, 100}; We have now created a variable that holds an array of four integers.
How an array is initialized and declared?
The initializer for an array is a comma-separated list of constant expressions enclosed in braces ( { } ). The initializer is preceded by an equal sign ( = ). You do not need to initialize all elements in an array.
How do you declare an array in a list?
Java ArrayList Example
- import java.util.*;
- public class ArrayListExample1{
- public static void main(String args[]){
- ArrayList<String> list=new ArrayList<String>();//Creating arraylist.
- list.add("Mango");//Adding object in arraylist.
- list.add("Apple");
- list.add("Banana");
- list.add("Grapes");
What are arrays and how is an array declared?
Arrays are used to store multiple values in a single variable, instead of declaring separate variables for each value. To create an array, define the data type (like int ) and specify the name of the array followed by square brackets [].
How to declare user defined array in Java?
We declare an array in Java as we do other variables, by providing a type and name: int[] myArray; To initialize or instantiate an array as we declare it, meaning we assign values as when we create the array, we can use the following shorthand syntax: int[] myArray = {13, 14, 15};
How do you declare and initialize an array?
Array Initialization Using a Loop
This is the most common way to initialize an array in C. // declare an array. int my_array[5]; // initialize array using a "for" loop.
How do you declare an array class?
We use the Class_Name followed by a square bracket [] then object reference name to create an Array of Objects. Class_Name[ ] objectArrayReference; Alternatively, we can also declare an Array of Objects as : Class_Name objectArrayReference[ ];
How declare array explain with example?
To create an array, define the data type (like int ) and specify the name of the array followed by square brackets []. To insert values to it, use a comma-separated list, inside curly braces: int myNumbers[] = {25, 50, 75, 100}; We have now created a variable that holds an array of four integers.
How to get array values in JavaScript?
- JavaScript Demo: Array.values()
- const array1 = ['a', 'b', 'c'];
- const iterator = array1. values();
-
- for (const value of iterator) {
- console. log(value);
- }
-
- // Expected output: "a"
How do you declare an array as a variable?
Procedure
- Define the DECLARE statement. Specify a name for the array data type variable. …
- Include the DECLARE statement within a supported context. This can be within a CREATE PROCEDURE, CREATE FUNCTION, or CREATE TRIGGER statement.
- Execute the statement which contains the DECLARE statement.
How to declare and initialize arrays explain with examples?
- We declare an array in Java as we do other variables, by providing a type and name: int[] myArray; To initialize or instantiate an array as we declare it, meaning we assign values as when we create the array, we can use the following shorthand syntax: int[] myArray = {13, 14, 15};
How do you declare and initiate an array?
There are two ways to specify initializers for arrays:
- With C89-style initializers, array elements must be initialized in subscript order.
- Using designated initializers, which allow you to specify the values of the subscript elements to be initialized, array elements can be initialized in any order.
How do you declare an array of variables?
Procedure
- Define the DECLARE statement. Specify a name for the array data type variable. …
- Include the DECLARE statement within a supported context. This can be within a CREATE PROCEDURE, CREATE FUNCTION, or CREATE TRIGGER statement.
- Execute the statement which contains the DECLARE statement.
How to declare array in JavaScript empty?
Assigning an array to a new empty array in JavaScript is the simplest and fastest technique for emptying arrays. n = []; In the above code, we simply assign array n to a new empty array.
How do you declare an array with variables?
To create array variables you must first create the array type and then declare the local array variable or create the global array variable. Creating an array data type is a task that you would perform as a prerequisite to creating a variable of the array data type.
What is array in Javascript with example?
An array is an object that can store multiple values at once. For example, const words = ['hello', 'world', 'welcome']; Here, words is an array. The array is storing 3 values.
How does array find work in JavaScript?
JavaScript Array find()
The find() method returns the value of the first element that passes a test. The find() method executes a function for each array element. The find() method returns undefined if no elements are found. The find() method does not execute the function for empty elements.